How to disable motor checks in multipurpose Pybricks programs
If you forget to plug in a motor that you use in your program, you normally see this tip to remind you, in the output window:
A sensor or motor is not connected to the specified port:
--> Check the cables to each motor and sensor.
--> Check the port settings in your script.
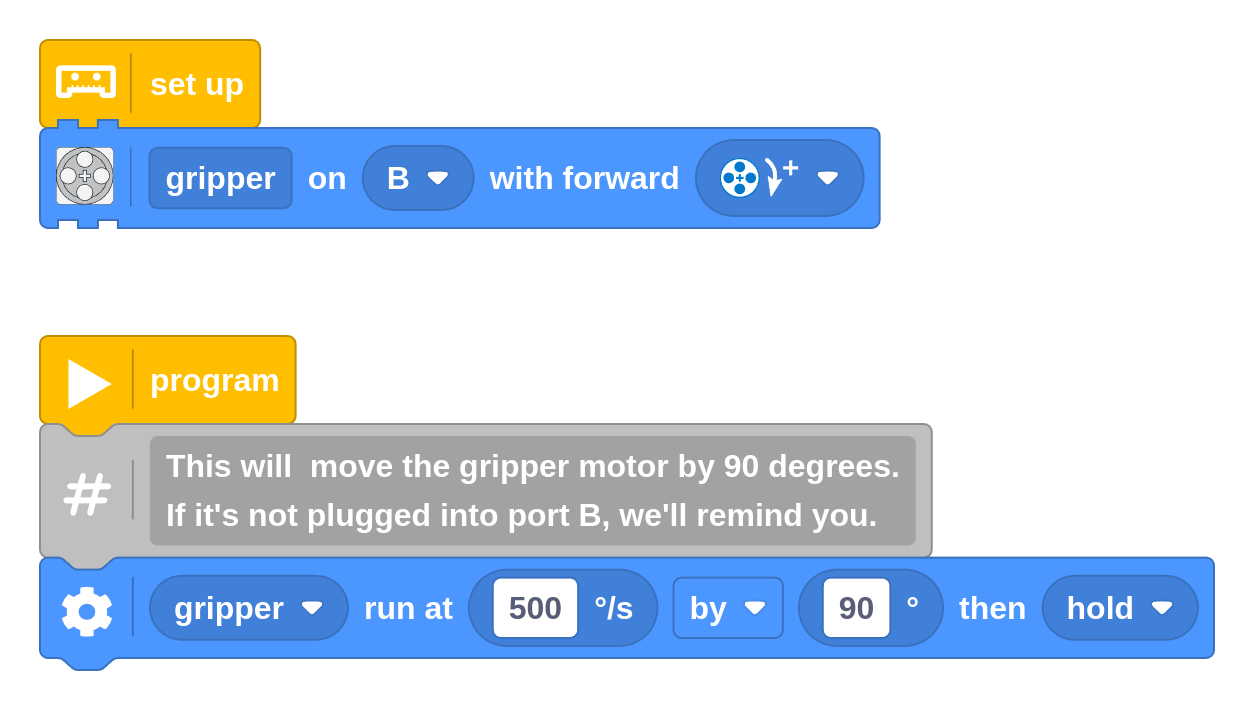
When coding, errors are not bad but they are meant to help you catch mistakes early on.
Why disable connection checks?
Most of the time, you’ll want to keep it that way. After all, now your robot won’t drive off your desk with one motor still going while the other isn’t plugged in (yep - we’ve all been there!)
But sometimes you just want to make a general purpose program that runs whether or not something is plugged in. For example, you might want to make a single program to test basic motion in all your creations.
In this article we’ll show you how to do this. You can do it with blocks or with Python!
Adding an extra module
To make this work, you’ll import an extra module that lets you override
the usual checks. To do that,
download the file called allow_missing_motors.py
and import it into the Pybricks editor so it
shows up under your files:
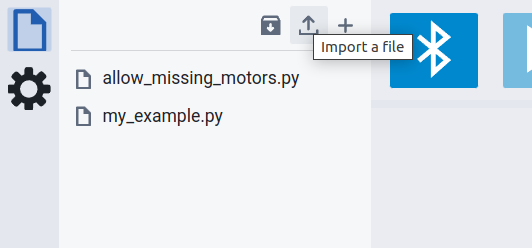
Because even block-based programs use Python under the hood, we can import this
module using the import
block from the external tasks
category. This makes it skips the usual checks:
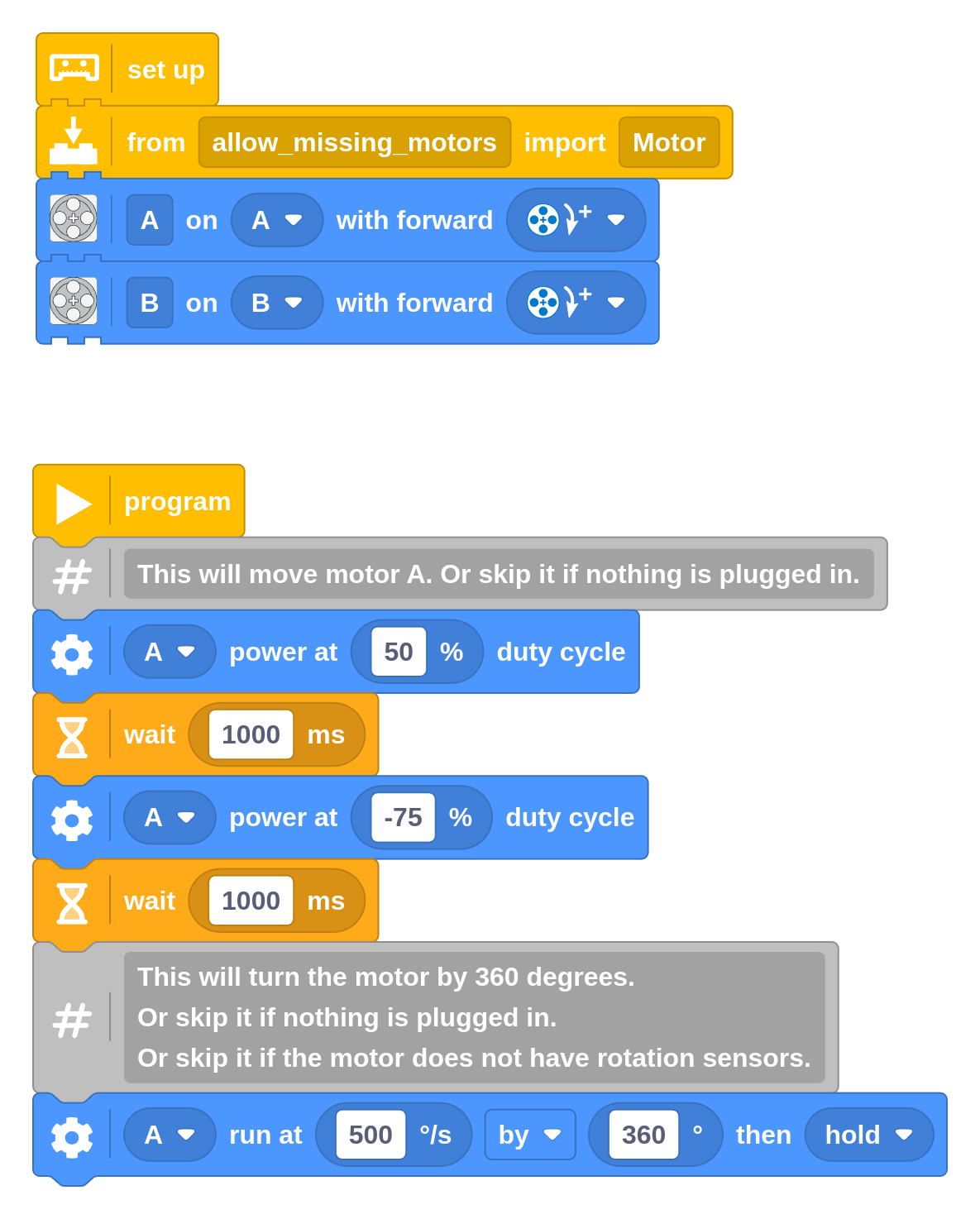
There’s no need to understand all the details in the Python module to use it. The main things to remember are:
- You only need to add import block once as shown in the example above.
- Only programs that use this import are affected. Every other program works as usual and will always check the ports.
- You can setup the motors at the top as usual. For lack of better names, you can just call them the same as the respective port.
- If a block you use is not supported by your motor, or nothing is plugged in, your program will just ignore that block instead of raising an error.
Making a simple battery box
Perhaps the simplest but useful example is to turn your hub into a simple battery box, as shown below.
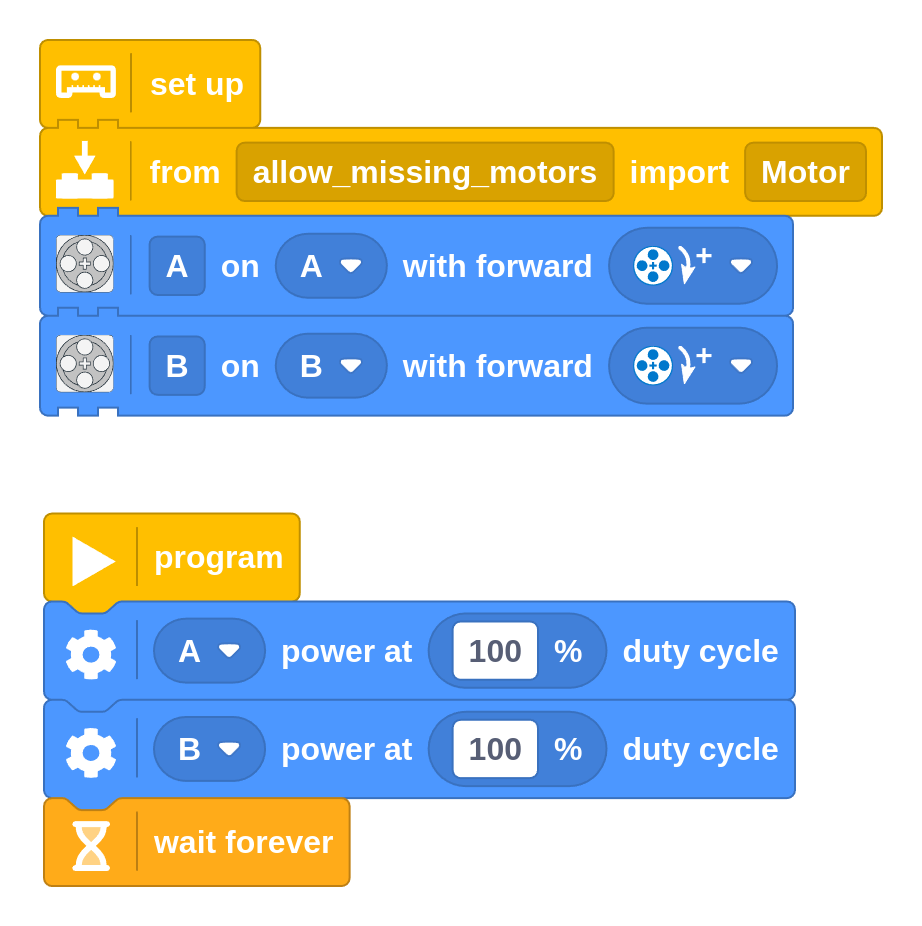
This just turns the motors on if they are connected. They keep running until you stop the program using the button on the hub.
As a result, you can start the motors by clicking the button, and click it again to turn them off. Just like a good old battery box!
Further exploration
With that extra module in place, you can easily get creative with your multipurpose script, no matter what is plugged in. Can you create a program that…
- … remote controls any of the ports?
- … controls the motors with the hub button?
- … changes the motor speed using the angle of motor?
Using Python for your main script
You can use this same technique if you use Python for your main program instead of blocks. If you look closely at the block program above, you can probably guess how this works. Just add the following line below your usual import statements. That’s it!
from allow_missing_motors import Motor