Train slope detection
Python code and building instructions for the LEGO City Cargo Train (60198).
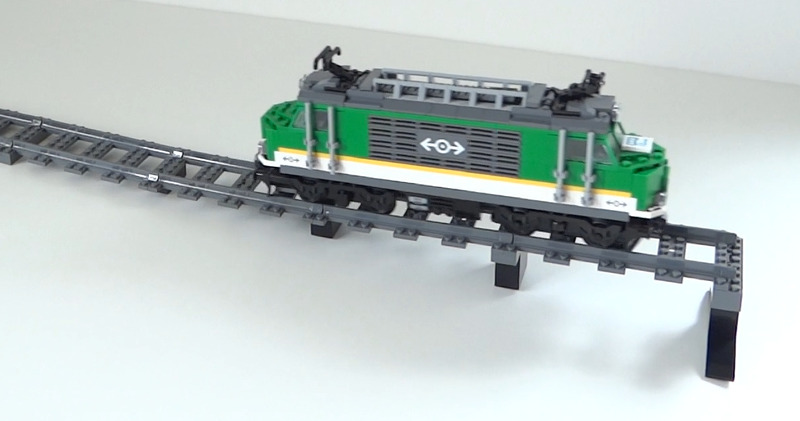
Detect the slope of the track. This lets you determine where the train is on the circuit.
Design modifications
Build the train using the standard instructions. Mount the tilt sensor horizontally in the train, with the cable side facing towards direction of driving:
Connect the motor to port A and connect the tilt sensor to port B.
Program
This program makes the train drive until it detects a slope of 8 degrees or more. Then it drives back down.
from pybricks.pupdevices import TiltSensor, DCMotor
from pybricks.parameters import Port
from pybricks.tools import wait
# Initialize the train motor.
# If you have a motor with encoders, use the Motor class instead.
train = DCMotor(Port.A)
# Initialize the tilt sensor.
sensor = TiltSensor(Port.B)
# Measure the tilt while the train is on a flat surface.
pitch_start, roll_start = sensor.tilt()
# Start driving.
train.dc(-50)
# We need to reach a constant speed before we can check tilt again,
# because acceleration affects tilt. So we wait a second.
wait(1000)
# Wait for the train to sense the hill.
while True:
pitch_now, roll_now = sensor.tilt()
wait(10)
# If we reached an extra 3 degrees, exit/break the loop.
if pitch_now >= pitch_start + 8:
break
# Drive backwards for a few seconds and then stop.
train.dc(40)
wait(3000)
train.dc(0)
Programming and running instructions
The train must start on a horizontal track. Then run the program.
Building instructions
- Building instructions part 1
- Building instructions part 2
- Building instructions part 3
- Building instructions part 4
- Building instructions part 5
- Building instructions part 6
This project was submitted by The Pybricks Team.